One of the most impressive controls of Visual Basic is the MSFlexGrid control. As you can guess by its name, the MSFlexGrid control is a descendant of theold Grid control. The MSFlexGrid control provides all the functionality for building spreadsheet applications, just as the RichTextBox control provides all the functionality for building word processing applications. Once you master its basic properties, writingspreadsheet- like applications for displaying data will just be a question of setting its properties.
The MSFlexGrid control is an extremely useful tool for displaying information in a tabular form. You can place it on your Forms to present nicely organized data to the user. Figure 9.9 shows a MSFlexGrid control displaying financial data. At first glance, it looks a lot like a spreadsheet: the first row and column are fixed and they contain titles, and the rest of the cells contain text (including numbers) or graphics arranged in rows and columns, which can vary in width and height. The scroll bars give the user easy access to any part of the control (although all the cells can scroll, the fixed cells always remain visible).
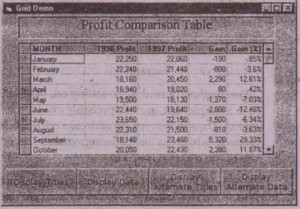
Despite external similarities, the MSFlexGrid control is not a complete spreadsheet in the same sense that the TextBox control is not a complete word processor, Although it can be the basis of a spreadsheet, this is not the purpose for which the MSFlexGrid control was designed. It is meant to display information in tabular form, but not for data entry.
Most of the properties of this control pertain to the placement of the data and their appearance; there are no properties or events for the immediate editing of the cells’ contents. However, it’s not too difficult to take control of the KeyPress event to capture keystrokes and add data entry capabilities to the control, as you’ll see later on in the section “The FlexGrid Application.”
Basic Properties
Let’s review the basic properties of the control by examining its operation. Recall that the grid’s cells can contain text, numbers, or images. You can assign a value ‘ to a cell in several ways, but all methods require that you first select the cell by means of its address on the grid and assign the desired value to its Text property.
‘The address of a cell is given by its Row and Cot properties, whose values start at 0. Row 0 is the fixed title row, and column 0 is the fixed title column. To address the first non-fixed cell in the grid of Figure 9.9, you would use the following statements:
Grid.Row = 1
Grid.Col = 1
After you specify the cell’s address, you can examine its contents with a statement like:
Cell Value = Grid.Text .
or set its contents with a statement like:
Grid.Text = “January”
This method of reading or setting cell values requires too many statements for such a simple task, and it’s probably available to be compatible with the old Grid control. The simplest way to address a cell on the grid is by means of the TextMatrix property, which has the following syntax :
Grid.TextMatrix(row, col)
The row and col arguments are the cell’s coordinates in the grid. To extract the value of the first editable cell, use’ a statement like:
Cell Value = Grid.TextMatrix(1, 1)
The names of the months and the corresponding profit figures of the grid shown in Figure 9.9 are assigned with the following loop, which is executed from within the Display Data button’s event handler:
Using the FormatString Property
Code 9.12:
The array MonthNames holds the names of the months and the arrays Profit 96 and Profit 97 contain the numeric values. Notice that the contents of the last two columns (Gain and Gain [%]) are calculated.
A third way to access the cells of the grid is by means of the TextArray property, which is similar to the Text Matrix property, but it uses a single index to address a cell. It has the following syntax: “
Grid.TextArray(cellindex)
To calculate the cellindex argument, which determines the location of the desired cell, multiply the desired row by the Cols property and add the desired. The following statement calculates the value of the cellindex arguments row (row) and column (001) number:
cellindex = row * cols + col
The TextArray property is less convenient than the TextMatrix property because you have to convert the actual address of the desired cell to a single number, Use the TextArray property to assign values to the grid’s cells if the values are already stored in a one-dimensional array. “